SOLID Software Design Principles helps to develop understandable, maintainable, and scalable software systems. Each letter of SOLID stands for one principle. The principles are a subset of many principles promoted by American software engineer and instructor Robert C. Martin
Design principles provide a proven solution for most common design problems and we only need to consider how we can transform our requirement to a solution with the components of these proven design principles.
“It is not enough for code to work”
Robert C. Martin
Application should be maintainable, understandable, and should be able to change with minimum effort without breaking existing functionalities.
What happens when we don’t follow design principles?
Code fragility – Tendency to break after changes.
Code rigidity – Difficult to change. Take a lot of time and effort to do a change.
Technical debt
The cost of prioritizing fast delivery over quality delivery for a long period of time
Fast delivery and Quality code are two different things that we can’t accommodate both at the same time.
If you prefer fast delivery in the early stage of the project, it will look effective. Fast delivery reduces the quality of the code. Hence in the long run, when you receive change requests, the time and effort to do even a simple change will cost you lots of time and effort. It won’t happen if you maintain a quality code from the beginning of the project.
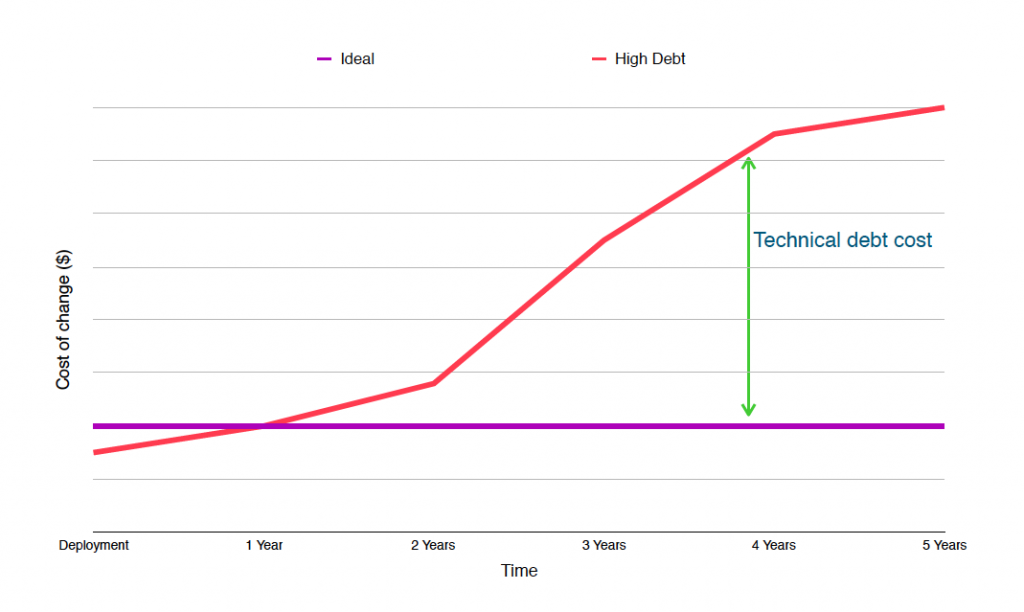
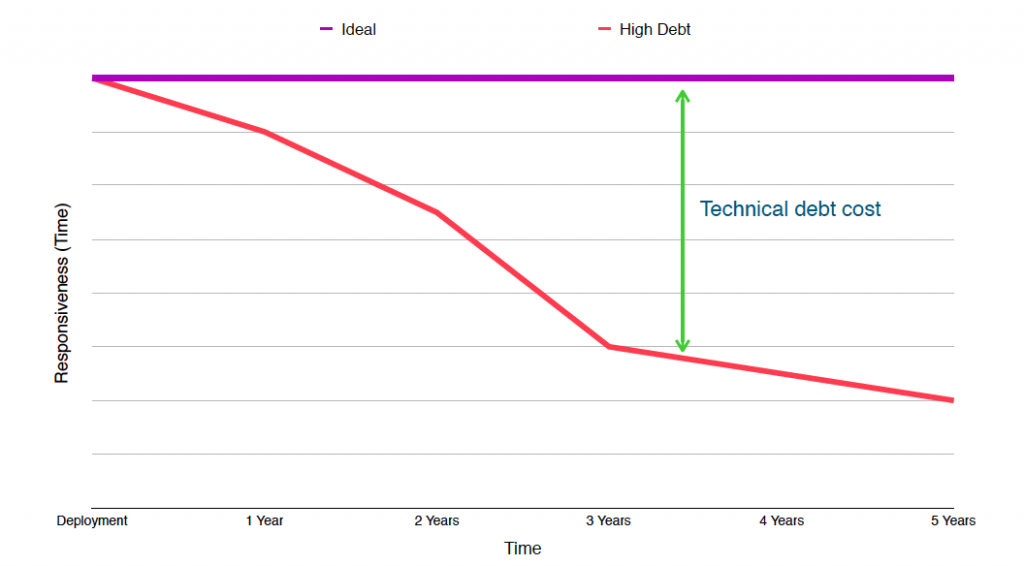
Fast delivery leads to increase technical debt
Quality delivery won’t increase the technical debt
Benefits of SOLID Software Design Principles
Clean code – easy to understand
Can change faster with minimal risk
Maintainable over long period of time
Cost effective
Alternative Methods to Improve Code Quality
Continues refactoring
Design patterns
Unit testing
SOLID Software Design Principles
Single Responsibility Principle
Open Closed Principle
Liskov Substitute Principle
Interface Segregation Principle
Dependency Inversion Principle
Single Responsibility Principle
The single responsibility principle states that one component(class, module, method or microservice) should have only one responsibility. It also says that there should be a single reason to change the component.
This doesn’t mean that the class should only have one method. This means that all the functions in a component should align with a single purpose.
For example, imagine a class where there are methods to place an order for a product and do the payment. If we need to change the ordering mechanism we need to change this class. If we need to change the payment mechanism we need to change this class. Placing an order and doing a payment are two very different causes to change this class. Having multiple reasons to change a single class can increase the danger of breaking the existing code.
By keeping these two tasks in two different components decrease the danger of breaking the existing functionalities. It made your components clean and easy to understand.
Open Closed Principle
Software entities should be open for extension but close for modification.
Modules, classes, or methods should be extendable without changing their behavior and should be able to add new features to the extended components.
By following this principle, new features can be added easily and with minimal cost. It also minimize the regression bug since it isolates the changes to a specific component.
Liskov Substitute Principle
This states that any object of a type must be substitutable by objects of a derived type without altering the correctness of that program. In other words, any implementation of an abstraction should be substitutable by any of the implementation of that particular abstraction.
We should clearly identify what the actual hierarchy and should eliminates falls hierarchies.
Interface Segregation Principle
An object should only depend on interfaces it requires and should not be forced to depend on methods or properties that they don’t require.
We need to identity FAT interfaces to break them into more focused lean interfaces. If an interface has lot of methods, if clients of that interface need to throw not implementing method exception or need to provide empty implementation and client forces to implement methods that are not relevant then you have a fat method that needs to be broken into pieces.
Dependency Inversion Principle
This states that high level modules should not depend on low level modules, both should depend on abstractions. Abstractions should not depend on details.
public class Gear{ } public class Engine{ Gear gear; } public class Car{ Engine engine; }
Class Car is tightly coupled with Engine class and Engine class is tightly coupled with Gear class. And this is a tightly coupled system. We can overcome this by introducing interfaces.
This principle improves loos coupling between components.
Conclusion
Writing great software is difficult. Even with the mature object-oriented principles, your program can be a mess. Doing so many things in a single code block, huge classes and methods, duplicate configurations are some common things that we face frequently. By using these proven solutions we can develop a great design.
Related articles :
i have visited this blog a couple of times now and i have to tell you that i find it quite nice actually. continue doing what youre doing! :p
Great goods from you, man. I have understand your stuff previous to and you are just extremely excellent. I actually like what youve acquired here, really like what you are saying and the way in which you say it. You make it entertaining and you still take care of to keep it smart. I cant wait to read much more from you. This is actually a great site.
Spot on with this write-up, I absolutely believe this website needs much more attention.
I’ll probably be back again to see more, thanks for the information!
Thanks for blogging this, it was unbelieveably informative and helped me tons
As it is curious.. 🙂
I really like when people are expressing their opinion and thought. So I like the way you are writing
Thank you for your post, and i suppose it helps me a lot.